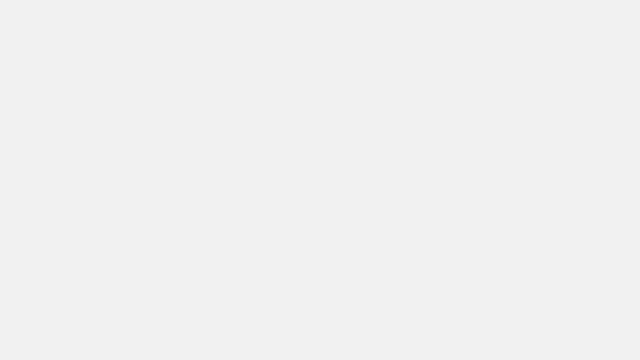
Night Light Development
Below are videos of my nightlight in action. The “simple” code simply fades the LED on and off in relation to the amount of light hitting the photoresistor. The “complex” code allows the user to freeze the LED using a button.
//delcaring variables for pins
const int fadeLed = 11;
const int potPin = 0;
const int lightPin = 1;
//time stuffs
unsigned long previousMillis = 0;
const long interval = 10;
//led fading
boolean fadeUP = true;
int fadeLevel = 0;
//led blinking
int ledState = LOW;
//analog sensors (pot & photores)
int lightVar = 0;
int potVar = 0;
//state variables
int stateVar;
void setup() {
pinMode(fadeLed, OUTPUT);
Serial.begin(9600);
}
void loop() {
boolean fadeUp = true;
// check time
unsigned long currentMillis = millis();
//dif functions for dif stateVar
switch (stateVar) {
case 0:
//check sensors
potVar = analogRead(potPin);
lightVar = analogRead(lightPin);
//logic
if (lightVar <= potVar) {
fadeUp = true;
} else {
fadeUp = false;
}
//display
if (currentMillis – previousMillis >= interval) {
previousMillis = currentMillis;
if (fadeUp == true) {
if (fadeLevel != 255) fadeLevel++;
} else {
if(fadeLevel != 0) fadeLevel–;
}
}
break;
case 2:
Serial.println(“night light mode”);
ledState= LOW;
stateVar = 0;
break;
}
//write to LEDs
analogWrite(fadeLed, fadeLevel);
}
//delcaring variables for pins
const int buttonPin = 2;
const int fadeLed = 11;
const int potPin = 0;
const int lightPin = 1;
//time stuffs
unsigned long previousMillis = 0;
const long interval = 10;
//led fading
boolean fadeUP = true;
int fadeLevel = 0;
//led blinking
int ledState = LOW;
//button stuff
int buttonState = 0;
int prevButtonState = LOW;
boolean pressed = false;
//analog sensors (pot & photores)
int lightVar = 0;
int potVar = 0;
//state variables
int stateVar;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(fadeLed, OUTPUT);
Serial.begin(9600);
}
void loop() {
boolean fadeUp = true;
// check time
unsigned long currentMillis = millis();
//check button
buttonState = digitalRead(buttonPin);
if (buttonState == HIGH && prevButtonState == LOW) {
delay(5);
stateVar++;
if (stateVar >= 4) stateVar = 0;
}
prevButtonState = buttonState;
//dif functions for dif stateVar
switch (stateVar) {
case 0:
//check sensors
potVar = analogRead(potPin);
lightVar = analogRead(lightPin);
//logic
if (lightVar <= potVar) {
fadeUp = true;
} else {
fadeUp = false;
}
//display
if (currentMillis – previousMillis >= interval) {
previousMillis = currentMillis;
if (fadeUp == true) {
if (fadeLevel != 255) fadeLevel++;
} else {
if(fadeLevel != 0) fadeLevel–;
}
}
break;
case 2:
Serial.println(“night light mode”);
ledState= LOW;
stateVar = 0;
break;
case 3:
Serial.println(“freeze light”);
fadeLevel = 0;
stateVar = 2;
case 4:
ledState = LOW;
break;
}
//write to LEDs
analogWrite(fadeLed, fadeLevel);
}